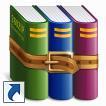
- Description
PRG 218 Week 5 Individual: Coding: Derived Classes
Create a base class and two derived classes. Also, create and call a member function named communicate() that behaves differently when called by each class. Create a single C++ project (and CPP source file) named animal_communication as follows:
- Into this source, type the source code for Program 15-16, “Program Output,” in Section 15.6, “Polymorphism and Virtual Member Functions,” in Ch. 15, “Inheritance, Polymorphism, and Virtual Functions,” of Starting Out With C++ From Control Structures Through Objects.
- Run and debug the code as necessary.
- Create a new class named Cat that derives from the base class Animal.
- In the main portion of the program, add code to instantiate the Cat class and telegraph that the Cat class’s constructor and destructor are being called.
Note: To do this, you may copy and customize the code that does this for the Dog class, choosing a variable name such as myAnimal2 for the instantiation of the Cat object.
- Create a member function called communicate() in the base Animal class, the Cat class, and the Dog class. The communicate() member function should produce “Speak” when called on an instance of Animal, “Woof!” when called on an instance of Dog, and “Meow!” when called on an instance of Cat as follows:
// Class member function for Animal
// Place public function below the constructor
// and destructor in the Animal class.
void communicate() {
cout << “Speak.” << endl;
}
// Class member function for Dog
// Place public function below the constructor
// and destructor in the Dog class.
void communicate() {
cout << “Woof!” << endl;
}
// Class member function for Cat
// Place public function below the constructor
// and destructor in the Cat class.
void communicate() {
cout << “Meow!” << endl;
}
- In the main portion of your program, instantiate the Animal, Cat, and Dog classes as shown below, and then call the communicate member function on each instance as follows:
int main() {
Animal genericAnimal;
genericAnimal.communicate();
Dog ralph;
ralph.communicate();
Cat fluffy;
fluffy.communicate();
return 0;
}
Save your C++ code in a file named Week5Program_YourName.cpp, and take a screenshot of your running program. Copy your screenshot in to Microsoft® Word document.
Zip both your screenshot document and CPP source into a single ZIP file named PRG218_Week5_YourName.zip.
Submit your ZIP file using the Assignment Files tab.